SAP UI5
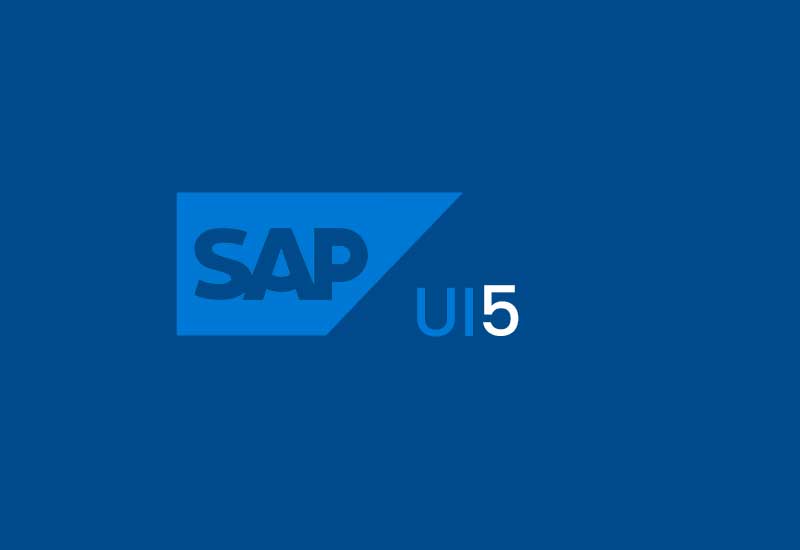
SAPUI5, FIORI , OData and SAP Net Weaver Gateway
SAPUI5:
- 1. SAP UX strategy and SAP Fiori UX.
- 2. Introduction to SAPUI5 and SAP FIORI and OData
- 3. Client and server architecture.
- 4. HTML/5 Basics:
- a. Getting started
- b. Tags, Attributes and Elements
- c. Page Titles
- d. Paragraphs, Headings
- e. Lists
- f. Links
- g. Images
- h. Forms
- i. Assignment on HTML
- 5. Introduction to Cascading Style Sheets (CSS)
- a. Applying CSS - Different ways you can apply CSS to HTML
- b. Selectors, Properties, and Values
- c. Colours
- d. Text
- e. Margins and Padding
- f. Borders
- g. Box Model in CSS
- h. Assignment on CSS
- 6. JavaScript
- a. JavaScript Basics
- i. The HTML DOM
- ii. JavaScript Syntax
- iii. Basic Rules
- iv. Accessing Elements
- 1. Dot Notation
- 2. Square Bracket Notation
- v. Where Is JavaScript Code Written?
- vi. JavaScript Objects, Methods and Properties
- 1. Methods
- 2. Properties
- vii. The Implicit window Object
- b. JavaScript Variables and their usage
- c. Working with Arrays
- d. Associative Arrays
- e. JavaScript Operators
- f. JavaScript Functions
- i. Global Functions
- 1. Number(object)
- 2. String(object)
- 3. isNaN(object)
- 4. parseFloat() and parseInt()
- ii. User-defined Functions
- 1. Function Declarations
- 2. Function Expressions
- 3. Passing Values to Functions
- 4. Returning Values from Functions
- 5. Variable Scope
- i. Global Functions
- g. Event Handlers
- h. Built-In JavaScript Objects
- i. String
- ii. Math
- iii. Date
- iv. The typeof Operator
- i. Conditionals and Loops
- i. Conditionals
- ii. if - else if - else Conditions
- iii. Switch / Case
- j. Loops
- i. while Loop Syntax
- ii. do...while Loop Syntax
- iii. for Loop Syntax
- iv. for...in Loop Syntax
- k. JavaScript Form Validation
- i. Accessing Form Data
- ii. Basics of Form Validation
- iii. Cleaner Validation
- iv. Validating Radio Buttons
- v. Validating Check Boxes
- l. CSS Object Model
- i. Changing CSS with JavaScript
- ii. Hiding and Showing Elements
- m. Scope in JavaScript
- n. Objects in JavaScript
- o. Object-Oriented Programming
- p. Prototyping in JavaScript
- q. JSON
- r. Assignment on JavaScript
- a. JavaScript Basics
- 7. Introduction to jQuery and AJAX
- 8. SAPUI5
- a. Environment setup for SAPUI5
- b. SAP WEB IDE and Eclipse
- c. SAPUI5 vs OPENUI5
- d. Bootstrapping
- e. Architecture of SAPUI5 Application
- f. Introduction to Controls in SAPUI5
- g. Controls API
- i. How to use controls properties, aggregations ,events and methods
- h. Different types of Containers and Layouts
- i. Coding Guidelines
- i. Code Formatting
- ii. Variable Naming Conventions
- j. Event handling in SAPUI5
- i. How to use simple Event Handler
- ii. How to use Event Information in Event handling.
- k. MVC (Model View Controller)
- i. Model
- ii. View
- iii. Controllers
- l. Types of Views
- m. View Instantiation and the Controller Lifecycle
- n. Types of Models
- i. JSON Model
- ii. ODATA Model
- iii. Resource model
- 9. Models and Binding
- a. Instantiation and Loading of Data
- b. Accessing Model values.
- c. Property Binding
- d. Element Binding
- e. Aggregation Binding
- f. Expression Binding and Calculated Fields
- g. Internationalization(I18n)
- h. How to apply filters and sorting.
- i. Custom formatters
- j. Fragments and Dialogs
- k. Lazy loading
- l. Types of Binding modes.
- m. Assignment on Basics of UI5 and Data binding
- 10. Routing and Navigation
- a. Routing Configuration
- b. Router Initialization
- c. Routing with and without parameters
- d. How to use EventBus
- e. Assignment on Routing
- 11. Mock Data and Mock Server Configuration
- 12. Performing CRUD operations in the sapui5 app.
- a. Create
- b. Read
- c. Update
- d. Remove
- 13. Debugging SAPUI5 Applications using Chrome Developer Tools
- a. Finding the data objects in controller files
- b. Tracing network calls in Network tab.
- 14. Improving the performance of an SAPUI5 Application.
- 15. SAPUI5 Application Unit testing with QUnit.
- a. Understanding Qunit
- b. Setting up the Qunit for unit testing sapui5 app
- c. Process of writing Qunit tests for formatters.
- d. Executing Qunit tests.
- 16. SAPUI5 Application Integration Testing using OPA5
- a. Understanding OPA5
- b. Setting up the OPA for Integration testing sapui5 app
- c. Process of writing OPA tests for different functionalities
- d. Executing OPA tests.
- 17. Assignment on SAPUI5
SAP Fiori:
- 1. Overview of Fiori
- 2. Fiori Design Guidelines
- 3. Introduction to SAP Fiori Launchpad
- a. End user perspective
- i. Exploring SAP Fiori Launchpad
- ii. Personalizing SAP Fiori
- a. End user perspective
- 4. Types of Fiori Apps
- a. Transactional apps
- b. Analytical apps
- c. Factsheet apps
- 5. Types of Deployment options and its pros and cons.
- 6. Fiori Architecture of different types of apps
- 7. Explaining the relationship between Roles, Catalogs, Groups and Apps
- 8. Standard Fiori Launchpad and Application Configuration
- a. Activating SAP FIori Launchpad FE and BE services.
- b. Creating SAP Fiori Groups
- c. Creating SAP Fiori Catalogs
- d. Creating LPD_CUST Target Mappings
- e. Creating SAPUI5 Target Mappings
- f. Creating Roles using PFCG
- 9. Customizing and extending Standard Fiori Apps in SAP WEB IDE.
- 10. Fiori Launchpad Designer vs Fiori Launchpad
- 11. UI Theme Designer
- 12. Configuring Custom SAPUI5 app in Fiori Launchpad
- 13. End to End SAPUI5 Application with Code Explanation
- 14. Interview Guidance – topics
OData and SAP NetWeaver Gateway
- 1. SAP Gateway Overview
- 2. OData Overview
- a. Introduction to REST
- b. Introduction to OData
- c. Performing OData operations
- d. Performing OData Queries
- 3. SAP Gateway Service Implementation
- a. Implementing a Gateway Service
- b. Defining a Data Model
- c. Implementing Read Operations
- d. Implementing Query Options
- e. Implementing Write Operations
- f. Consuming OData Service
- g. Performing CRUD operations in Gateway client.
- h. Registering and activating a service